C# ToString explained
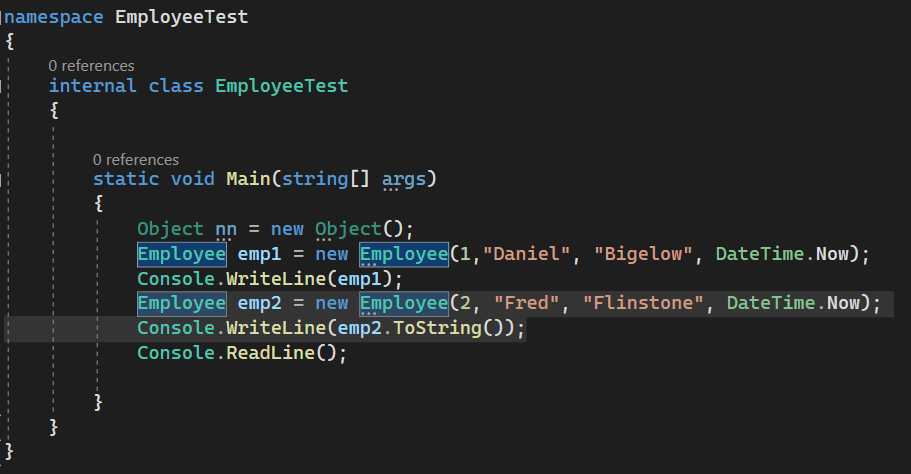
Let's say that we are creating a new object called "Employee" that will house an employee's data. Here we can store things like employee ID, First Name, Last Name, and employment date. We can then use these employee objects in other classes and methods.
This type of thing is a common part of object-oriented programming. A class created in C# is implicitly a child that is a type of Object inherently. This means that all methods that belong to the base C# object are also accessible from the classes that we create.
One of these inherited properties is called toString. By default toString prints the name of the class that is being called.
Employee emp2 = new Employee(2, "Fred", "Flinstone", DateTime.Now);
Console.WriteLine(emp2.ToString());
As an example, this code would print the name of the namespace in my case EmployeeTest followed by the class name of the employee. So "EmployeeTest.Employee" would be printed. This behavior is so built into c# that we can even shorten our code here.
Employee emp2 = new Employee(2, "Fred", "Flinstone", DateTime.Now);
Console.WriteLine(emp2);
Changing emp2.ToString() to emp2 will also print a string as it will assume that we want to convert emp2 to a string when being used as a parameter that accepts strings.
Printing the name of a method isn't super useful, I have never run into a need for this to happen. This is where Overrides come into play with our classes. With this, we can override the ToString method of the base Object class.
Here is my class Employee class that Overrides the ToString Method. In this case I have overridden the ToString method with one that prints the Last Name a comma a space, and then the First Name.
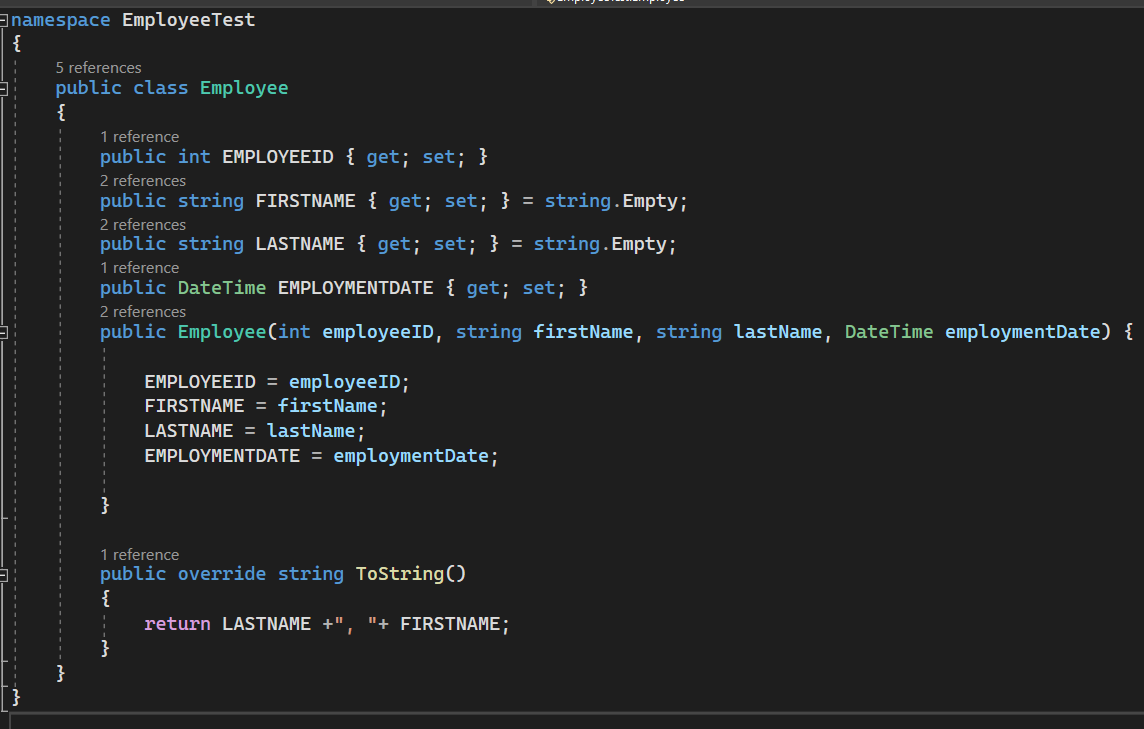
This will mean that any time I use Employee as a string, or call the ToString method, I will print the last name and first name instead of the class name.
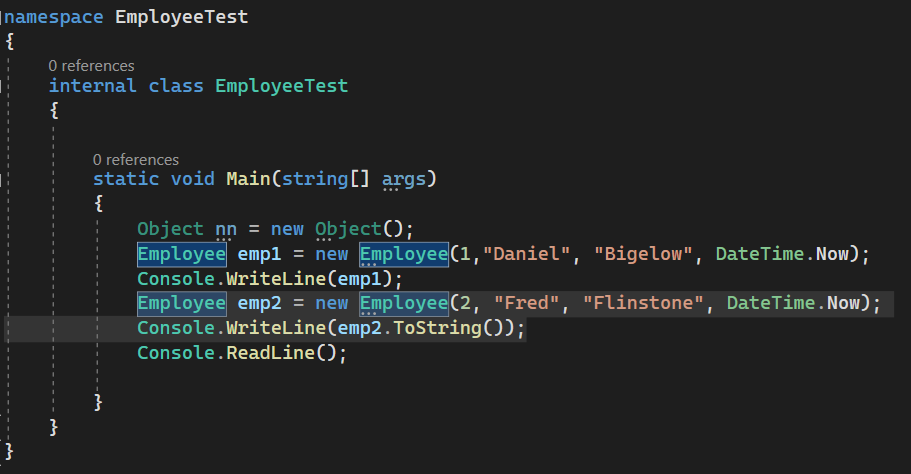
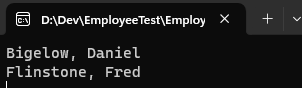
This can be useful in a lot of situations and becomes vital when creating UI items like drop-down lists. Overriding the ToString method is fairly common in coding, and luckily it's easy to do.